2023. 6. 20. 22:44ㆍFront/JS
1. Event (이벤트)
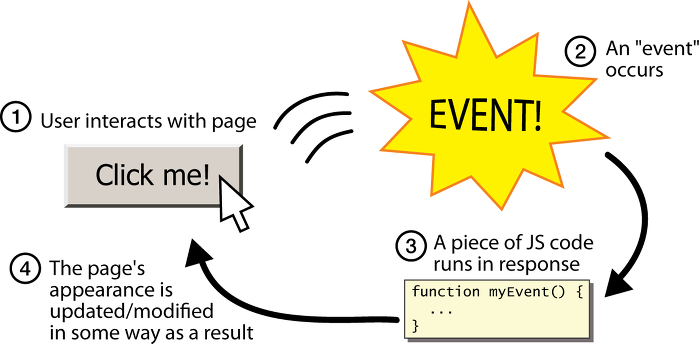
- 이벤트는 '사건'
- 브라우저에서의 사건(event)이란
사용자가 클릭을 했을 '때', 스크롤을 했을 '때', 필드의 내용을 바꾸었을 '때'와 같은 것을 의미한다.
2. Event 종류
1) Mouse Event
- click : 마우스 클릭
- dbclick : 마우스 더블클릭
- mousedown : 마우스 버튼을 누르고 있을 때
- mouseup : 누르고 있던 마우스 버튼을 해제할 때
- mousemove : 마우스를 움질일 때 (터치X)
- mouseover : 어떤 요소 위에 마우스가 위치할 때 (터치X)
- mouseout : 어떤 요소밖으로 마우스가 벗어날 때 (터치X)
2) Keyboard Event
- keydown : 키를 누르고 있을 때
- keypress : 키를 누르고 있을 때 (키보드를 누르고 있을 때 계속 실행됨)
- keyup : 누르고 있던 키를 해제할 때
3) Form Event
- focus / focusin : 요소가 포커스를 얻었을 때
- blur / focusout : 요소가 포커스를 잃었을 때
- change : value값이 변경되었을 때
- submit : form태그를 action의 주소로 전송
HTML DOM Event Object
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
www.w3schools.com
3. Event 등록 (Event Handler)
1) inline 방식
<태그이름 on이벤트명 = "사용할 함수 호출; 사용할 함수 호출2 ..">
2) property 방식
var id = document.getElementById("아이디명") ;
id.on이벤트명 = function(){ } ;
3) addEventListener 방식
var id = document.getElementById("아이디명") ;
id.addEventListener("이벤트명", 실행할 함수);
inline 방식 예시
<input type="button" value="CLICK" onclick="go(); go2()">
// 같은 이벤트로 여러개 함수 호출 가능 (세미콜론 사용해서)
<script type="text/javascript">
function go() {
alert('CLICK');
}
function go2() {
alert('CLICK2');
}
</script>
property 방식 예시
<select>
<option class="opt" selected ="selected">KT</option>
<option class="opt">SKT</option>
<option class="opt">LG</option>
</select>
<button id="btn"> CLICK </button>
<script type="text/javascript">
var btn = document.getElementById("btn");
btn.onclick = function() { // 같은 이벤트로 여러개 함수 호출 불가능
var opt = document.getElementsByClassName("opt");
for(i=0;i<opt.length;i++){
if(opt[i].selected){
alert(opt[i].innerHTML);
break;
}
}
</script>
addEventListener 방식 예시
<h2>ALL</h2>
<input type="checkbox" class="chk">
<h3>First</h3>
<input type="checkbox" class="chk">
<h3>Second</h3>
<input type="checkbox" class="chk">
<h3>Third</h3>
<input type="checkbox" class="chk">
<button id="btn">JOIN</button>
<script type="text/javascript">
var btn = document.getElementById("btn");
var chk = document.getElementsByClassName("chk");
chk[0].addEventListener("click", function(){
if(chk[0].checked){
for(i=1;i<chk.length;i++){
chk[i].checked=true;
}
} else {
for(i=1;i<chk.length;i++){
chk[i].checked=false;
}
}
});
btn.addEventListener("click", function(){
var cnt = 0;
for(i=1;i<chk.length;i++){
if(chk[i].checked) cnt++;
if(cnt==chk.length-1) alert("회원 가입을 축하합니다");
else alert("약관에 모두 동의해야 가입이 가능합니다");
}
});
</script>
4. Event 전파
- 중첩된 요소들은 부모와 자식 간의 관계를 갖는다 (예: div 안에 또다른 div)
- 부모와 자식 간에 같은 이벤트가 등록되어 있을 때, 자식의 이벤트가 발생하면 부모로 이벤트가 전달된다.
1) EVENT.preventDefault()
- 태그나 폼에 submit을 클릭하면 페이지가 이동한다
- 요소가 가지고있는 기본 동작을 중단시키기 위해 사용
2) EVENT.stopPropagation()
- 자식의 이벤트가 부모 요소로 전파되는 것을 중단시키기 위해 사용
<a href="https://www.naver.com/" id="d1">GO NAVER</a>
<script type="text/javascript">
var d1 = document.getElementById("d1");
d1.addEventListener("click", function(e){
console.log("GO NAVER");
e.preventDefault(); //웹사이트로 이동하는 것을 중단시킴
});
</script>
<form id="joinForm">
<input type="text" id="name"><br>
<input type="submit" value="회원가입">
</form>
<script>
var f = document.getElementById("joinForm");
f.addEventListener("submit", function(e){
if(f.name.value.length==0){
alert("이름를 입력하세요");
e.preventDefault(); //submit되지 않도록 동작중단
f.name.focus();
return;
}
}
</script>
5. Event 강제 실행
- 다른 요소의 설정된 이벤트를 강제로 실행하고 싶을 때 사용하는 방법
- 선택자.이벤트명()